Thoughts on Vibe Coding and Cursor
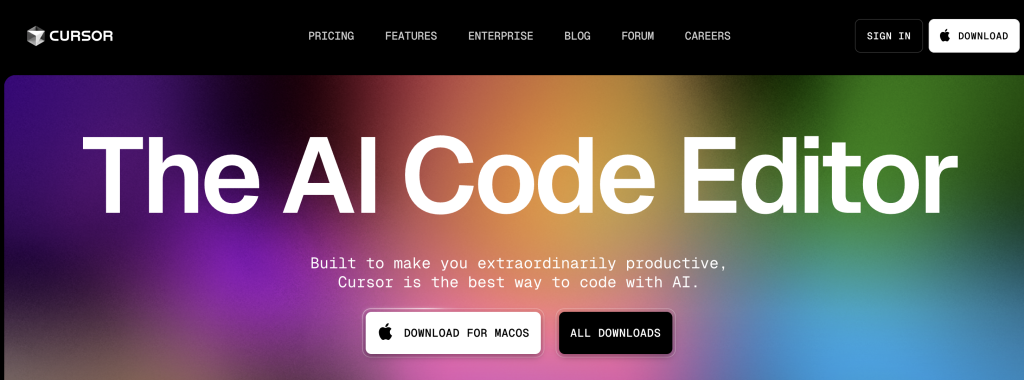
I’ve spent 10 hours a day the past couple of weeks vibe coding my SaaS as well as several other projects you can see on my GitHub. During that time I have learned a lot about Cursor and decided to document my thoughts here. There’s certainly more to it than just asking Claude “plz fix”.
In this blog post, we’ll explore the art and science of vibe coding with Cursor, focusing on best practices that can help you maximize productivity, creativity, and code quality. We’ll also examine how to effectively use Grok 3 as a technical lead while leveraging Claude for implementation—a powerful combination that can supercharge your development workflow.
What is Vibe Coding?
Vibe coding represents a fundamental shift in how we approach software development. Rather than starting with rigid specifications and diving immediately into implementation details, vibe coding begins with a high-level description of what you want to build—the “vibe” or essence of your project. The AI then helps translate this vision into working code, allowing for a more natural and intuitive development process.
This approach is particularly powerful because it allows developers to focus on the creative and strategic aspects of programming while letting AI handle much of the mechanical implementation. It’s not about replacing developers but augmenting their capabilities and allowing them to work at a higher level of abstraction.
A couple of tips for Cursor (an IDE)
1. Create a .cursorrules File
A .cursorrules
file in your project root directory provides project-specific instructions to the AI. This is crucial for consistent vibe coding results. Here’s a template to start with:
You are an expert developer with deep knowledge of [YOUR_TECH_STACK].
Code Style and Structure:
- Write clean, maintainable code with appropriate comments
- Follow [YOUR_CODING_STANDARDS]
- Prioritize readability and simplicity over clever solutions
- Use consistent naming conventions
- Structure code logically with clear separation of concerns
Project Context:
[BRIEF_DESCRIPTION_OF_YOUR_PROJECT]
When generating code:
- Consider performance implications
- Include error handling
- Write with testability in mind
- Consider edge cases
Customize this template to reflect your project’s specific requirements and coding standards.
2. Set Up Notepads for Key Concepts
Cursor’s Notepads feature is invaluable for vibe coding. Create notepads for:
- Project architecture overview
- Key design patterns and principles
- Common code snippets and patterns
- API documentation and examples
- User stories or requirements
These notepads can be referenced during your vibe coding sessions, providing important context to the AI.
The Dual-Model Workflow: Grok 3 as Technical Lead, Claude as Implementer
One of the most powerful approaches to vibe coding is using multiple AI models in a coordinated workflow. Here’s how to effectively use Grok 3 as your technical lead while leveraging Claude for implementation:
Understanding the Strengths of Each Model
Grok 3 excels at:
- High-level architectural thinking
- Strategic planning and system design
- Creative problem-solving
- Exploring multiple approaches
- Identifying potential issues and edge cases
Claude excels at:
- Precise code implementation
- Following specific instructions
- Maintaining consistency with existing code
- Detailed documentation
- Explaining implementation decisions
Implementing the Dual-Model Workflow
Step 1: Vision and Planning with Grok 3
Begin by describing your project or feature to Grok 3 in broad terms:
I want to build a real-time collaborative document editor with version history, user presence indicators, and commenting functionality. What would be the best approach?
Ask Grok 3 to:
- Outline multiple potential approaches
- Identify key technical challenges
- Recommend technology choices
- Sketch a high-level architecture
Step 2: Technical Specification with Grok 3
Once you have a high-level plan, ask Grok 3 to create a more detailed technical specification:
Based on your recommendation to use a CRDT-based approach with WebSockets, can you create a detailed technical plan? Include component structure, data models, and key algorithms we'll need to implement.
The goal is to get a comprehensive technical plan that can guide implementation.
Step 3: Implementation with Claude in Cursor
With your technical plan in hand, switch to Cursor and use Claude for implementation:
- Share the technical plan from Grok 3 with Claude
- Break down implementation into manageable chunks
- Ask Claude to implement specific components:
Based on the technical plan, let's implement the CRDT data structure for tracking document changes. It should support concurrent edits, track authorship, and be serializable for transmission over WebSockets.
Step 4: Iterative Refinement
As you build your application, continue this iterative process:
- Use Grok 3 to solve architectural challenges and make high-level decisions
- Use Claude in Cursor to implement the specific code
- Test and validate each component
- Return to Grok 3 when you encounter strategic challenges
This workflow leverages the strengths of each model: Grok 3’s strategic thinking and Claude’s implementation capabilities.
Advanced Prompting Techniques for Vibe Coding
The quality of your vibe coding experience depends significantly on how you communicate with the AI. Here are advanced prompting techniques specifically tailored for Cursor:
1. Set the Scene with Context
Before diving into specific requests, provide context about what you’re building and why:
I'm building a healthcare scheduling system that needs to handle complex appointment rules, recurring appointments, and provider availability. The system will be used by administrative staff who aren't technically sophisticated. Let's focus on creating an intuitive interface for setting up recurring appointment patterns.
2. Use the “Think, Plan, Execute” Pattern
Structure complex prompts in three distinct phases:
Let's think through how to implement a drag-and-drop interface for rearranging appointments.
First, THINK about the key challenges and considerations for this feature.
Then, PLAN the implementation approach, considering libraries, event handling, and state management.
Finally, EXECUTE by writing the code for the core drag-and-drop functionality.
This pattern encourages the AI to reason through problems methodically rather than jumping straight to implementation.
3. Leverage Role-Based Prompting
Assign specific roles to guide the AI’s perspective:
I want you to act as a security expert reviewing this authentication implementation. Identify potential vulnerabilities and suggest improvements to make it more secure.
Or:
Take on the role of a senior UX designer. Review this form component and suggest improvements to make it more user-friendly and accessible.
4. Use Iterative Refinement
Start with a basic implementation and refine it through conversation:
Let's create a basic data visualization component using D3.js.
Then iterate:
Now let's enhance it with interactive tooltips and animations.
And further:
Finally, let's optimize it for performance and add responsive behavior for different screen sizes.
5. Explicitly Request Alternatives
When you’re not sure about the best approach, ask for multiple options:
Show me three different ways we could implement this user authentication system. For each approach, explain the pros and cons, and recommend which one would be best for our specific needs.
6. Use “Vibe Descriptions” for UI Components
For UI elements, describe the feeling or experience you want to create:
Create a landing page hero section that feels energetic and modern, with bold typography, subtle animations, and a gradient background that suggests innovation and creativity.
Context Management: The Key to Effective Vibe Coding
Perhaps the most critical aspect of successful vibe coding is effective context management. Here are best practices specifically for Cursor:
1. Strategic File Selection
Don’t try to give the AI your entire codebase. Instead:
- Use
@
to add only the most relevant files to the context - Keep key files open in your editor
- Reference specific files with
/
when asking questions
For example:
I'm working on implementing the shopping cart functionality. I have the @ShoppingCart.tsx component open, and I need to integrate it with the @useCart.ts hook. How should I modify the component to display the cart items and total?
2. Use the “Context Window” Wisely
Cursor has a context limit (approximately 20,000 tokens). To use it effectively:
- Start new chats for new topics or features
- Remove irrelevant files from context when switching topics
- Prioritize recent and relevant code over older or peripheral code
3. Create Context-Rich Notepads
Use Notepads to store important context that you can reference across multiple conversations:
- System architecture diagrams
- API specifications
- Design patterns and coding standards
- User stories and requirements
Reference these notepads using the @
syntax when needed.
4. Leverage Cursor’s Context Finding
Cursor can automatically find relevant context in your codebase. Use prompts like:
Find all code related to user authentication in my project and help me understand how it works.
Or:
I need to modify how we handle form validation. Can you find the relevant files and explain the current implementation?
Maintaining Code Quality in Vibe Coding
One concern with AI-assisted coding is maintaining high code quality. Here are best practices to ensure your vibe-coded projects remain maintainable and robust:
1. Always Review Generated Code
Never accept generated code without review. Look for:
- Logical errors or bugs
- Security vulnerabilities
- Performance issues
- Consistency with your coding standards
- Proper error handling
2. Request Explanations
Ask the AI to explain complex or unfamiliar code it generates:
Can you explain how this reducer function works and why you chose this particular implementation?
3. Enforce Testing
Make testing an integral part of your vibe coding workflow:
Now that we've implemented the authentication service, let's write comprehensive unit tests for it, covering both successful authentication and various failure scenarios.
4. Use the “Refactor for Simplicity” Pattern
After implementing functionality, always ask for simplification:
The code works, but it seems more complex than necessary. Can you refactor it to be more concise and readable while maintaining the same functionality?
5. Implement Progressive Enhancement
Start with minimal viable implementations and enhance them progressively:
- First, get basic functionality working
- Then add error handling and edge cases
- Next, optimize for performance
- Finally, enhance with additional features
This approach ensures you have working code at each step rather than trying to build everything at once.
Real-World Vibe Coding Patterns
Let’s explore some practical patterns for vibe coding in different scenarios:
Pattern 1: The Feature Expansion
Start with a minimal feature description and progressively expand it:
Let's create a user profile page that displays basic user information.
Then:
Now let's add the ability for users to edit their profile information.
And:
Let's implement profile picture uploads with preview and cropping functionality.
Finally:
Let's add validation for all form fields and proper error handling.
Pattern 2: The Architectural Exploration
Use vibe coding to explore different architectural approaches:
I'm building a data-intensive dashboard application. What are the different architectural approaches I could take, and what are the trade-offs?
Then dive deeper into the chosen approach:
The micro-frontend approach sounds promising. How would I structure the project, and what technologies would work best for this approach?
Pattern 3: The Code Archaeologist
Use vibe coding to understand and improve existing code:
I'm trying to understand this legacy authentication system. Can you help me analyze how it works and identify areas for improvement?
Then:
Based on your analysis, let's refactor the authentication system to use modern best practices while maintaining backward compatibility.
Pattern 4: The Learning Accelerator
Use vibe coding to learn new technologies or frameworks:
I want to learn React Server Components. Can you create a simple application that demonstrates the key concepts and best practices?
Then:
Explain how the code you've written showcases the benefits of React Server Components and how it differs from traditional React applications.
Common Pitfalls and How to Avoid Them
Even with best practices, there are common pitfalls in vibe coding. Here’s how to avoid them:
Pitfall 1: Over-Reliance on AI
Symptoms:
- Accepting code without understanding it
- Inability to debug or modify code without AI assistance
- Declining personal coding skills
Solution:
- Challenge yourself to understand all generated code
- Regularly write code without AI assistance
- Use AI as a learning tool, not just a code generator
Pitfall 2: Context Overload
Symptoms:
- AI responses become generic or irrelevant
- Confusion between different parts of your project
- Inconsistent code generation
Solution:
- Start new chats for new topics
- Be selective about what files you include in context
- Use clear, focused prompts
Pitfall 3: Vague Prompts
Symptoms:
- AI generates code that doesn’t meet your needs
- Multiple iterations required for simple tasks
- Inconsistent results
Solution:
- Be specific about requirements and constraints
- Provide examples when possible
- Break complex requests into smaller, clearer prompts
Pitfall 4: Ignoring Edge Cases
Symptoms:
- Code works for the happy path but fails in edge cases
- Production bugs in AI-generated code
- Technical debt accumulation
Solution:
- Explicitly ask about edge cases
- Request error handling for all generated code
- Test thoroughly, including boundary conditions
Conclusion: The Future of Vibe Coding
Vibe coding with Cursor represents a fundamental shift in how we approach software development. By leveraging the strengths of AI models like Grok 3 and Claude, developers can focus on the creative and strategic aspects of programming while accelerating implementation.
As AI coding tools continue to evolve, the line between high-level concepts and implementation details will blur further, enabling even more intuitive and productive development workflows. By embracing vibe coding today, you’re not just adopting a new tool—you’re preparing for the future of software development.
Remember that the goal of vibe coding isn’t to replace human developers but to augment their capabilities, allowing them to work at a higher level of abstraction and focus on the aspects of development that truly require human creativity and judgment. With Cursor as your partner, you can ride the wave of this technological revolution and create better software faster than ever before.